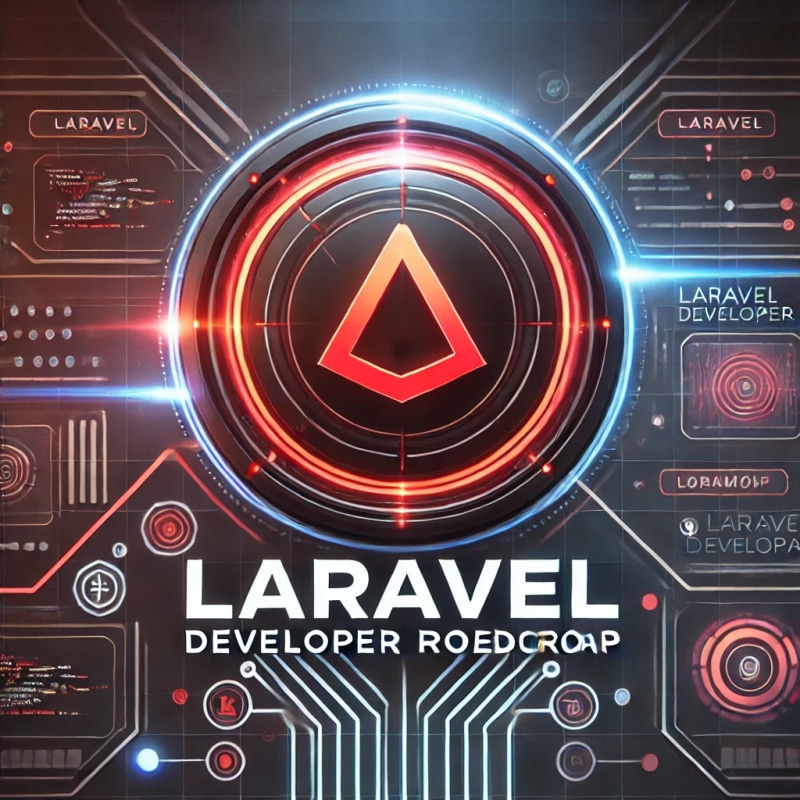
PHP Fundamentals
- OOP Concepts: Encapsulation, Inheritance, Polymorphism, Abstraction
- SOLID Principles: Single Responsibility, Open-Closed, Liskov, Interface Segregation, Dependency Inversion
- Dependency Injection & Service Container
- Composer: Autoloading, Managing Dependencies
Routing & Middleware
- Route Model Binding: Implicit & Explicit
- Middleware: Custom Middleware, Authentication, Role-Based Access
- Rate Limiting & Throttle Requests
- API Routing Best Practices
Blade Templating
- Blade Components & Slots
- Layouts & Sections for Reusability
- Blade Directives:
@foreach
, @if
, @auth
, @can
- Custom Blade Directives
Authentication & Authorization
- Laravel Sanctum: Token-Based Authentication
- Laravel Passport: OAuth Authentication
- Policies & Gates: Role-Based Access Control (RBAC)
- Social Authentication: Laravel Socialite
Database & Eloquent ORM
- Migrations & Schema Design
- Seeders & Factories: Generating Dummy Data
- Relationships: One-to-One, One-to-Many, Many-to-Many, Polymorphic
- Query Scopes for Reusable Queries
Advanced Eloquent
- Eager Loading vs Lazy Loading
- Query Optimization with Indexing
- Using Observers for Model Events
- Mutators & Accessors for Data Formatting
File Handling & Storage
- Uploading & Managing Files (Local & Cloud)
- Spatie Medialibrary for Media Management
- Secure File Downloads & Pre-Signed URLs
REST API Development
- API Resources & Transformers
- Error Handling & HTTP Status Codes
- API Documentation: Swagger / Scribe
- Rate Limiting & Token Expiration
Testing
- Unit & Feature Tests with PHPUnit
- PEST for Concise Testing
- Mocking & Faking Dependencies
- Browser Testing with Laravel Dusk
- Redis & Memcached for Data Caching
- Queues & Workers for Background Tasks
- Optimizing Query Performance & Route Caching
Task Scheduling & Queues
- Laravel Scheduler for Automating Commands
- Job Chaining & Failed Job Handling
- Queue Workers & Prioritization
Real-Time Features
- Broadcasting Events & Notifications
- WebSockets with Laravel Echo & Pusher
- Implementing Real-Time Chat & Live Updates
Security Best Practices
- CSRF Protection & Middleware
- Preventing XSS & SQL Injection
- Content Security Policies & Secure Headers
- Rate Limiting & API Security
Multi-Tenancy & Scalable Architectures
- Multiple Database Connections
- Sharding & Load Balancing
- Managing Multi-Tenant Applications
CI/CD for Laravel
- Automated Deployments using GitHub Actions
- Deploying with Envoyer & Deployer
- Managing Environment Variables & Secrets
Laravel Ecosystem
- Livewire for Reactive Components
- Inertia.js for Vue.js/React.js Integration
- Jetstream, Fortify, & Filament for Authentication
- Laravel Nova for Admin Panels
Microservices & API-First Architecture
- GraphQL APIs in Laravel
- Event-Driven Laravel with RabbitMQ/Kafka
- Running Laravel with Octane for High Performance
- Profiling with Laravel Telescope
- Debugging Queries with Ray & Laravel Debugbar
- Database Query Optimization & Indexing
State Management for Full-Stack Apps
- Vuex for Vue.js Applications
- Redux/Zustand for React.js State Management
Payments & Subscription Systems
- Laravel Cashier for Subscription Billing
- Stripe & PayPal Integrations
- Handling Webhooks for Payment Processing
Infrastructure & DevOps for Laravel
- Dockerizing Laravel for Development & Production
- Kubernetes for Laravel Microservices
- Managing Background Jobs with Supervisor
- Deploying Laravel on AWS (EC2, S3, RDS, Lambda)